Now Available:
Computer Programming: An Introduction for the Scientifically InclinedSander Stoks
ISBN: 978-90-812788-1-2
Paperback, 284 pages — now also available as e-book!
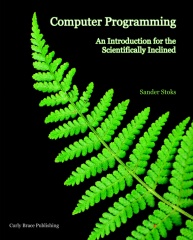
Computers are used in many areas of science. Basic programming skills are taught in virtually every science course today. However, these courses are often still somewhat of a "second class citizen" at many science faculties, and most "beginner level" programming books do not focus on scientific use.
This book presents the basics of computer programming in a way that satisfies the particular needs of science students, while at the same time clarifying concepts from a software engineering and computer science point of view.
No prior programming experience is required to read and understand this book. It starts "from scratch". Creating and running programs on Windows, Linux, and Mac OS X is covered.
The language used in this book is C, the "lingua franca" of computer programming. It covers ANSI C and C99. With chapters on computer graphics (including how to generate your own) and scientific simulations, it presents material which is directly useful to the science student.
Also includes an overview of available libraries, where to find them, and how to use them in your own programs.
The full table of contents is listed below. A three-chapter excerpt (chapters 1, 2, and 9) of the e-book version can be downloaded from here (note that this excerpt is specifically formatted for small screen digital e-book reading devices, so the page layout is different from the paperback version.) An HTML version of Chapter 1 is available online here.
0 Introduction
0.1 Intended Audience
0.2 Why Another Programming Book?
0.3 Assumed Prior Knowledge
0.4 How to Read This Book
0.5 Scope of This Book
0.6 Acknowledgements
1 Computers
1.1 Do You Need To Know?
1.2 Hardware Overview
1.2.1 Processor
1.2.2 Memory
1.2.3 Peripherals and Interfaces
1.2.4 Networks and Clustering
1.3 Binary Arithmetic
1.3.1 Negative Values
1.3.2 Floating Point Numbers
1.3.3 Range and Accuracy
1.3.4 Rational and Complex Numbers
1.4 Operating Systems
1.5 Specialized Machines
1.6 Synopsis
1.7 Questions and Exercises
2 Programs
2.1 Computer Recipes
2.2 A Real Program
2.3 The Same Program in C
2.4 Running Your Programs
2.4.1 Building on Windows
2.4.2 Building on UNIX Systems
2.4.3 Running Your Hello World
2.5 Programming Languages
2.5.1 Variations in C
2.6 Errors and Warnings
2.7 Synopsis
2.8 Questions and Exercises
3 Functions and Variables
3.1 Hello World, Again
3.2 Variables and Types
3.3 Expressions
3.4 Functions
3.4.1 Format Strings
3.5 Prototypes
3.6 Into the Void
3.7 Names
3.8 Comments
3.9 More about Math
3.10 Return Values and Error Codes
3.11 Macros
3.12 The Main Thing
3.13 Synopsis
3.14 Other Languages
3.15 Questions and Exercises
4 Program Flow
4.1 Decisions
4.2 Switches
4.3 Boolean Expressions
4.4 Loops
4.5 Caveats
4.6 The Ternary Operator
4.7 Scope
4.8 A Useful Example: Roots
4.8.1 Speeding Things Up
4.8.2 The Order of an Algorithm
4.9 Recursion
4.10 Synopsis
4.11 Other Languages
4.12 Questions and Exercises
5 Arrays, Pointers, and Memory
5.1 Arrays
5.2 Bounds
5.3 Strings
5.3.1 Encoding
5.3.2 Termination
5.3.3 String Manipulation
5.4 Multi-Dimensional Arrays
5.5 Pointers
5.6 Returning Arrays From Functions
5.7 Dynamic Memory Allocation
5.8 Passing Arrays To Functions
5.9 A Useful Example: Factors
5.10 Variable Length Arrays
5.11 Synopsis
5.12 Other Languages
5.13 Questions and Exercises
6 Data Types and Structures
6.1 Levels of Abstraction
6.2 Type Definitions
6.3 Data Structures
6.3.1 "Fake" Strong Typing
6.3.2 Call-by-Name vs. Call-by-Value
6.3.3 Heterogeneous Structures
6.3.4 Complex Data Structures
6.3.5 Alternatives to Arrays
6.4 Synopsis
6.5 Other Languages
6.6 Questions and Exercises
7 Files
7.1 Storage Devices
7.2 File Systems
7.3 File I/O in C
7.4 Structure of Data in Files
7.4.1 Human-Readable Files
7.4.2 Binary Files
7.4.3 File Formats
7.4.4 Random Access
7.5 A Useful Example: Least Squares Fitting
7.5.1 Theory
7.5.2 Implementation
7.6 Redirection
7.7 Synopsis
7.8 Other Languages
7.9 Questions and Exercises
8 Bits and Shifts
8.1 Bitwise Operators
8.2 3D Puzzles
8.2.1 Introduction
8.2.2 Solving Strategy
8.2.3 Permutations
8.2.4 Data Type and Manipulation
8.2.5 Fitting It All Together
8.3 Intermezzo: Optimization
8.3.1 Compiler Switches
8.3.2 Avoiding Calculations
8.3.3 Minor Optimizations
8.4 (Very) Basic Cryptography
8.4.1 The Caesar Shift Cipher
8.4.2 The Vigenre Cipher
8.5 Precedence
8.6 Synopsis
8.7 Other Languages
8.8 Questions and Exercises
9 Simulations
9.1 Virtual Experiments
9.2 A Pendulum
9.2.1 Analytical Derivation
9.2.2 Numerical Simulation
9.2.3 Extending the Simulation
9.3 Random Values
9.4 Percolation
9.5 Synopsis
9.6 Other Languages
9.7 Questions and Exercises
10 Complex Projects
10.1 Multi-File Programs
10.2 Header Files Revisited
10.2.1 Your Own Header File
10.2.2 Error Prevention
10.2.3 Multiple Inclusions
10.2.4 Intermezzo: Conditional Compilation
10.2.5 Header Guards
10.3 Translation Units
10.4 Building Revisited
10.4.1 Separate Compilation
10.4.2 Dependencies
10.4.3 Automated Build Tools
10.4.4 Include Paths
10.5 Libraries
10.6 Callbacks and Function Pointers
10.7 Synopsis
10.8 Other Languages
10.9 Questions and Exercises
11 Graphics
11.1 Graphical User Interfaces
11.2 Visualization vs. Interpretation
11.3 Vectors and Bitmaps
11.3.1 GUIs Revisited
11.3.2 Image files
11.4 Two Useful Examples
11.4.1 Dithering
11.4.2 The Mandelbrot Set
11.5 Synopsis
11.6 Other Languages
11.7 Questions and Exercises
12 Libraries
12.1 Library Mechanics
12.1.1 Static and Shared Libraries
12.1.2 Installing Libraries
12.1.3 Versioning
12.2 A Word on Licenses
12.3 The Standard C Library
12.3.1 Math
12.3.2 Input/Output
12.3.3 String Handling
12.3.4 Date and Time
12.3.5 General Utilities
12.4 Using the Operating System
12.4.1 Cross-Platform Development
12.5 Numerical Libraries
12.5.1 BLAS and LAPACK
12.5.2 Numerical Recipes
12.5.3 NAG
12.5.4 GSL
12.5.5 FFTW
12.5.6 Processor-Specific Libraries
12.6 Miscellaneous
12.7 Synopsis
12.8 Other Languages
12.9 Questions and Exercises
Bibliography